从尾到头打印链表 - 数据结构 - 机器学习
1140 人阅读 | 时间:2021年01月15日 01:22
数据结构 - 机器学习
深度学习
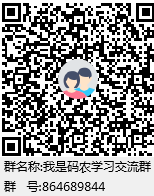
当前位置:首页 » 面试笔试 » 正文
从尾到头打印链表
12260 人参与 2019年03月19日 17:09 分类 : 面试笔试 评论
题目描述
输入一个链表,按链表值从尾到头的顺序返回一个ArrayList。
有三种思路,第一就是利用栈先入后出的特性完成,第二就是存下来然后进行数组翻转。
第三是利用递归。
栈思路:
class Solution { public: vector<int> printListFromTailToHead(ListNode* head) { vector<int> value; ListNode *p=NULL; p=head; stack<int> stk; while(p!=NULL){ stk.push(p->val); p=p->next; } while(!stk.empty()){ value.push_back(stk.top()); stk.pop(); } return value; } };
数组翻转:数组翻转可以用C++自带的函数,也可以自己实现
class Solution { public: vector<int> printListFromTailToHead(ListNode* head) { vector<int> value; ListNode *p=NULL; p=head; while(p!=NULL){ value.push_back(p->val); p=p->next; } //reverse(value.begin(),value.end()); //C++自带的翻转函数 int temp=0; int i=0,j=value.size()-1; while(i<j){ temp=value[i]; //也可以用swap函数,swap(value[i],value[j]); value[i]=value[j]; value[j]=temp; i++; j--; } return value; } };
递归思路:
class Solution { public: vector<int> value; vector<int> printListFromTailToHead(ListNode* head) { ListNode *p=NULL; p=head; if(p!=NULL){ if(p->next!=NULL){ printListFromTailToHead(p->next); } value.push_back(p->val); } return value; } };
其他:
最佳代码:代码思路借助栈,遍历的时候入栈,由于数据结构中栈的特点是先进后出,所以遍历的过程中压栈,推栈,完了弹栈加到ArrayList中。有两个容易出错的地方:第一,第一次测试用例,{}返回[
],null是null,而[ ]是new ArrayList()但是没有数据。第二,遍历stack用的方法是!stak.isEmpty()方法,而不是for循环size遍历。。
/**
* public class ListNode {
* int val;
* ListNode next = null;
*
* ListNode(int val) {
* this.val = val;
* }
* }
*
*/
import java.util.Stack;
import java.util.ArrayList;
public class Solution {
public ArrayList<Integer>
printListFromTailToHead(ListNode listNode) {
if(listNode == null){
ArrayList list = new ArrayList();
return list;
}
Stack<Integer> stk = new Stack<Integer>();
while(listNode != null){
stk.push(listNode.val);
listNode = listNode.next;
}
ArrayList<Integer> arr = new ArrayList<Integer>();
while(!stk.isEmpty()){
arr.add(stk.pop());
}
return arr;
}
}
来源:我是码农,转载请保留出处和链接!
本文链接:http://www.54manong.com/?id=1234
(function() {
var s = "_" + Math.random().toString(36).slice(2);
document.write('');
(window.slotbydup = window.slotbydup || []).push({
id: "u3646208",
container: s
});
})();
(function() {
var s = "_" + Math.random().toString(36).slice(2);
document.write('');
(window.slotbydup = window.slotbydup || []).push({
id: "u3646147",
container: s
});
})();
微信号:qq444848023 QQ号:444848023
加入【我是码农】QQ群:864689844(加群验证:我是码农)
- 不用加减乘除做加法2019-03-20 19:09
- 把二叉树打印成多行2019-03-19 12:42
- 滑动窗口的最大值2019-03-20 11:10
- 程序员面试题-变态跳台阶问题2019-03-14 11:02
(function() {
var s = "_" + Math.random().toString(36).slice(2);
document.write('');
(window.slotbydup = window.slotbydup || []).push({
id: "u3646186",
container: s
});
})();
(function() {
var s = "_" + Math.random().toString(36).slice(2);
document.write('');
(window.slotbydup = window.slotbydup || []).push({
id: "u3646175",
container: s
});
})();
搜索
网站分类
- 数据结构
- 数据结构视频教程
- 数据结构练习题
- 数据结构试卷
- 数据结构习题解析
- 数据结构电子书
- 数据结构精品文章
- 区块链
- 区块链精品文章
- 区块链电子书
- 大数据
- 大数据精品文章
- 大数据电子书
- 机器学习
- 机器学习精品文章
- 机器学习电子书
- 面试笔试
- 物联网/云计算
标签列表
- 数据结构 (39)
- 数据结构电子书 (20)
- 数据结构习题解析 (8)
- 数据结构试卷 (10)
- 区块链是什么 (261)
- 数据结构视频教程 (31)
- 大数据技术与应用 (12)
- 百面机器学习 (14)
- 机器学电子书 (29)
- 大数据电子书 (37)
- 程序员面试 (10)
- RFID (21)
最近发表
- 找出数组中有3个出现一次的数字
- 《百面机器学习》电子书下载
- 区块链精品电子书《深度探索区块链:Hyperledger技术与应用_区块链技术丛书》张增骏
- 区块链精品电子书《比特币:一个虚幻而真实的金融世界》
- 区块链精品电子书《图说区块链》-徐明星 & 田颖 & 李霁月
- 区块链精品电子书《是非区块链:技术、投机与泡沫》-英国《金融时报》
- 区块链精品电子书《商业区块链:开启加密经济新时代》-威廉·穆贾雅
- 区块链精品电子书《人工智能时代,一本书读懂区块链金融 (互联网_时代企业管理实战系列)》-马兆林
-
(function(){
var bp = document.createElement('script');
var curProtocol = window.location.protocol.split(':')[0];
if (curProtocol === 'https'){
bp.src = 'https://zz.bdstatic.com/linksubmit/push.js';
}
else{
bp.src = 'http://push.zhanzhang.baidu.com/push.js';
}
var s = document.getElementsByTagName("script")[0];
s.parentNode.insertBefore(bp, s);
})();
全站首页 | 数据结构 | 区块链| 大数据 | 机器学习 | 物联网和云计算 | 面试笔试
var cnzz_protocol = (("https:" == document.location.protocol) ? "https://" : "http://");document.write(unescape("%3Cspan id='cnzz_stat_icon_1276413723'%3E%3C/span%3E%3Cscript src='" + cnzz_protocol + "s23.cnzz.com/z_stat.php%3Fid%3D1276413723%26show%3Dpic1' type='text/javascript'%3E%3C/script%3E"));本站资源大部分来自互联网,版权归原作者所有!
©著作权归作者所有:来自ZhiKuGroup博客作者没文化的原创作品,如需转载,请注明出处,否则将追究法律责任
来源:ZhiKuGroup博客,欢迎分享。
评论专区